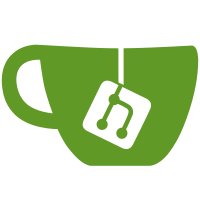
The performance improvement from this will not be noticable (perhaps there isn't one), however it is part of the bigger plan to move all of the DB access to ContentProviders. This will make a big improvement to the startup time of the app, given we are currently loading all of the apps to populate the list of apps. It will come at the cost of some apparantly weird code convensions. Most notably, when loading data from a content provder, you only ask for the fields that you intend to use. As a result of my Helper class which converts results from the content providers cursor into Repo value objects, there is no guarantee that certain attributes will be available on the value object. E.g. if I load repos and only ask for "_ID" and "ADDRESS", then it is meaningless to ask the resulting Repo object for its "VERSION" (it wont be there), despite it being a perfectly legal attribute from the Java compilers perspective. Repo.id field has also been made private (sqlite is the only entity should be able to set id's), and made id a long (sqlite stores identifiers as longs rather than ints).
235 lines
9.2 KiB
XML
235 lines
9.2 KiB
XML
<?xml version="1.0" encoding="utf-8"?>
|
|
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
|
|
package="org.fdroid.fdroid"
|
|
android:installLocation="auto"
|
|
android:versionCode="580"
|
|
android:versionName="0.58" >
|
|
|
|
<uses-sdk
|
|
android:minSdkVersion="5"
|
|
android:targetSdkVersion="19" />
|
|
|
|
<supports-screens
|
|
android:anyDensity="true"
|
|
android:largeScreens="true"
|
|
android:normalScreens="true"
|
|
android:resizeable="true"
|
|
android:smallScreens="true"
|
|
android:xlargeScreens="true" />
|
|
|
|
<uses-feature
|
|
android:name="android.hardware.touchscreen"
|
|
android:required="false" />
|
|
|
|
<uses-permission android:name="android.permission.INTERNET" />
|
|
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
|
|
<uses-permission android:name="android.permission.RECEIVE_BOOT_COMPLETED" />
|
|
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
|
|
|
|
<application
|
|
android:name="FDroidApp"
|
|
android:icon="@drawable/ic_launcher"
|
|
android:label="@string/app_name"
|
|
android:allowBackup="true"
|
|
android:theme="@style/AppThemeDark"
|
|
android:supportsRtl="false" >
|
|
|
|
<provider
|
|
android:authorities="org.fdroid.fdroid.data"
|
|
android:name="org.fdroid.fdroid.data.RepoProvider"
|
|
android:exported="false"/>
|
|
|
|
<activity
|
|
android:name=".FDroid"
|
|
android:configChanges="keyboardHidden|orientation|screenSize" >
|
|
|
|
<intent-filter>
|
|
<action android:name="android.intent.action.MAIN" />
|
|
|
|
<category android:name="android.intent.category.LAUNCHER" />
|
|
</intent-filter>
|
|
|
|
<intent-filter>
|
|
<action android:name="android.intent.action.VIEW" />
|
|
|
|
<category android:name="android.intent.category.DEFAULT" />
|
|
<category android:name="android.intent.category.BROWSABLE" />
|
|
|
|
<data android:scheme="http" />
|
|
<data android:scheme="https" />
|
|
<data android:host="f-droid.org" />
|
|
<data android:host="www.f-droid.org" />
|
|
<data android:pathPrefix="/repository/browse" />
|
|
</intent-filter>
|
|
|
|
<meta-data
|
|
android:name="android.app.default_searchable"
|
|
android:value=".SearchResults" />
|
|
</activity>
|
|
<activity
|
|
android:name=".ManageRepo"
|
|
android:allowTaskReparenting="true"
|
|
android:label="@string/menu_manage"
|
|
android:launchMode="singleTop"
|
|
android:parentActivityName=".FDroid" >
|
|
<meta-data
|
|
android:name="android.support.PARENT_ACTIVITY"
|
|
android:value=".FDroid" />
|
|
|
|
<intent-filter>
|
|
<action android:name="android.intent.action.VIEW" />
|
|
|
|
<category android:name="android.intent.category.BROWSABLE" />
|
|
<category android:name="android.intent.category.DEFAULT" />
|
|
|
|
<data android:scheme="http" />
|
|
<data android:scheme="https" />
|
|
<data android:host="*" />
|
|
<!--
|
|
The pattern matcher here is poorly implemented, in particular the * is
|
|
non-greedy, so you have to do stupid tricks to match patterns that have
|
|
repeat characters in them. http://stackoverflow.com/a/8599921/306864
|
|
-->
|
|
<data android:path="/fdroid/repo" />
|
|
<data android:pathPattern="/fdroid/repo/*" />
|
|
<data android:pathPattern="/.*/fdroid/repo" />
|
|
<data android:pathPattern="/.*/fdroid/repo/*" />
|
|
<data android:pathPattern="/.*/.*/fdroid/repo" />
|
|
<data android:pathPattern="/.*/.*/fdroid/repo/*" />
|
|
<data android:pathPattern="/.*/.*/.*/fdroid/repo" />
|
|
<data android:pathPattern="/.*/.*/.*/fdroid/repo/*" />
|
|
<data android:path="/fdroid/archive" />
|
|
<data android:pathPattern="/fdroid/archive/*" />
|
|
<data android:pathPattern="/.*/fdroid/archive" />
|
|
<data android:pathPattern="/.*/fdroid/archive/*" />
|
|
<data android:pathPattern="/.*/.*/fdroid/archive" />
|
|
<data android:pathPattern="/.*/.*/fdroid/archive/*" />
|
|
<data android:pathPattern="/.*/.*/.*/fdroid/archive" />
|
|
<data android:pathPattern="/.*/.*/.*/fdroid/archive/*" />
|
|
</intent-filter>
|
|
<intent-filter>
|
|
<action android:name="android.intent.action.VIEW" />
|
|
|
|
<category android:name="android.intent.category.BROWSABLE" />
|
|
<category android:name="android.intent.category.DEFAULT" />
|
|
|
|
<data android:scheme="fdroidrepo" />
|
|
<data android:scheme="fdroidrepos" />
|
|
</intent-filter>
|
|
</activity>
|
|
|
|
<activity
|
|
android:name=".views.RepoDetailsActivity"
|
|
android:label="@string/menu_manage"
|
|
android:parentActivityName=".ManageRepo" />
|
|
|
|
<activity
|
|
android:name=".AppDetails"
|
|
android:label="@string/app_details"
|
|
android:exported="true"
|
|
android:parentActivityName=".FDroid" >
|
|
<meta-data
|
|
android:name="android.support.PARENT_ACTIVITY"
|
|
android:value=".FDroid" />
|
|
|
|
<intent-filter>
|
|
<action android:name="android.intent.action.VIEW" />
|
|
|
|
<category android:name="android.intent.category.DEFAULT" />
|
|
<category android:name="android.intent.category.BROWSABLE" />
|
|
|
|
<data android:scheme="fdroid.app" />
|
|
</intent-filter>
|
|
|
|
<intent-filter>
|
|
<action android:name="android.intent.action.VIEW" />
|
|
|
|
<category android:name="android.intent.category.DEFAULT" />
|
|
<category android:name="android.intent.category.BROWSABLE" />
|
|
|
|
<data android:scheme="market" android:host="details" />
|
|
</intent-filter>
|
|
|
|
<intent-filter>
|
|
<action android:name="android.intent.action.VIEW" />
|
|
|
|
<category android:name="android.intent.category.DEFAULT" />
|
|
<category android:name="android.intent.category.BROWSABLE" />
|
|
|
|
<data android:scheme="http" />
|
|
<data android:scheme="https" />
|
|
<data android:host="f-droid.org" />
|
|
<data android:host="www.f-droid.org" />
|
|
<data android:pathPrefix="/app/" />
|
|
</intent-filter>
|
|
</activity>
|
|
<activity
|
|
android:label="@string/menu_preferences"
|
|
android:name=".PreferencesActivity"
|
|
android:parentActivityName=".FDroid" >
|
|
<meta-data
|
|
android:name="android.support.PARENT_ACTIVITY"
|
|
android:value=".FDroid" />
|
|
</activity>
|
|
<activity
|
|
android:name=".SearchResults"
|
|
android:label="@string/search_results"
|
|
android:exported="true"
|
|
android:launchMode="singleTop"
|
|
android:parentActivityName=".FDroid" >
|
|
<meta-data
|
|
android:name="android.support.PARENT_ACTIVITY"
|
|
android:value=".FDroid" />
|
|
|
|
<intent-filter>
|
|
<action android:name="android.intent.action.SEARCH" />
|
|
</intent-filter>
|
|
|
|
<intent-filter>
|
|
<action android:name="android.intent.action.VIEW" />
|
|
|
|
<category android:name="android.intent.category.DEFAULT" />
|
|
<category android:name="android.intent.category.BROWSABLE" />
|
|
|
|
<data android:scheme="fdroid.search" />
|
|
</intent-filter>
|
|
|
|
<intent-filter>
|
|
<action android:name="android.intent.action.VIEW" />
|
|
|
|
<category android:name="android.intent.category.DEFAULT" />
|
|
<category android:name="android.intent.category.BROWSABLE" />
|
|
|
|
<data android:scheme="market" android:host="search" />
|
|
</intent-filter>
|
|
|
|
<meta-data
|
|
android:name="android.app.searchable"
|
|
android:resource="@xml/searchable" />
|
|
</activity>
|
|
|
|
<!--Used for SSL TOFU, supported by extern/MemorizingTrustManager lib -->
|
|
<activity android:name="de.duenndns.ssl.MemorizingActivity" />
|
|
|
|
<receiver android:name=".StartupReceiver" >
|
|
<intent-filter>
|
|
<action android:name="android.intent.action.BOOT_COMPLETED" />
|
|
|
|
<category android:name="android.intent.category.HOME" />
|
|
</intent-filter>
|
|
</receiver>
|
|
<receiver android:name=".PackageReceiver" >
|
|
<intent-filter>
|
|
<action android:name="android.intent.action.PACKAGE_ADDED" />
|
|
<action android:name="android.intent.action.PACKAGE_UPGRADED" />
|
|
<action android:name="android.intent.action.PACKAGE_REMOVED" />
|
|
|
|
<data android:scheme="package" />
|
|
</intent-filter>
|
|
</receiver>
|
|
|
|
<service android:name=".UpdateService" />
|
|
</application>
|
|
|
|
</manifest>
|